1 | # react-typeahead
|
2 |
|
3 | > A typeahead/autocomplete component for React
|
4 |
|
5 | react-typeahead is a javascript library that provides a react-based
|
6 | typeahead, or autocomplete text entry, as well as a "typeahead tokenizer",
|
7 | a typeahead that allows you to select multiple results.
|
8 |
|
9 | ## Usage
|
10 |
|
11 | For a typeahead input:
|
12 |
|
13 | ```javascript
|
14 | var Typeahead = require('react-typeahead').Typeahead;
|
15 | React.render(
|
16 | <Typeahead
|
17 | options={['John', 'Paul', 'George', 'Ringo']}
|
18 | maxVisible= 2
|
19 | />
|
20 | );
|
21 | ```
|
22 |
|
23 | For a tokenizer typeahead input:
|
24 |
|
25 | ```javascript
|
26 | var Tokenizer = require('react-typeahead').Tokenizer;
|
27 | React.render(
|
28 | <Tokenizer
|
29 | options={['John', 'Paul', 'George', 'Ringo']}
|
30 | onTokenAdd={function(token) {
|
31 | console.log('token added: ', token);
|
32 | }}
|
33 | />
|
34 | );
|
35 | ```
|
36 |
|
37 | ## Examples
|
38 |
|
39 | * [Basic Typeahead with Topcoat][1]
|
40 | * [Typeahead Tokenizer with Topcoat][2]
|
41 | * [Typeahead Tokenizer with simple styling][3]
|
42 |
|
43 | 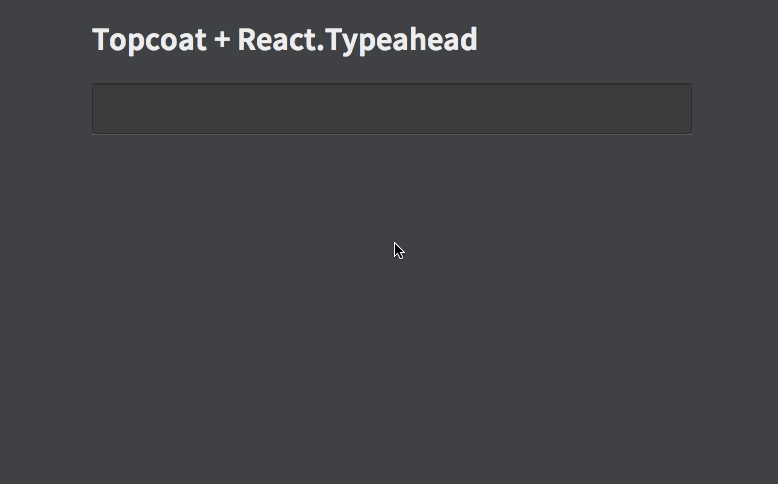
|
44 |
|
45 | [1]: http://wookiehangover.github.com/react-typeahead/examples/typeahead-topcoat.html
|
46 | [2]: http://wookiehangover.github.com/react-typeahead/examples/tokenizer-topcoat.html
|
47 | [3]: http://wookiehangover.github.com/react-typeahead/examples/TypeaheadTokenizer-simple.html
|
48 | [4]: http://blog.npmjs.org/post/85484771375/how-to-install-npm
|
49 |
|
50 | ## API
|
51 |
|
52 | ### Typeahead(props)
|
53 |
|
54 | Type: React Component
|
55 |
|
56 | Basic typeahead input and results list.
|
57 |
|
58 | #### props.options
|
59 |
|
60 | Type: `Array`
|
61 | Default: []
|
62 |
|
63 | An array supplied to the filtering function.
|
64 |
|
65 | #### props.maxVisible
|
66 |
|
67 | Type: `Number`
|
68 |
|
69 | Limit the number of options rendered in the results list.
|
70 |
|
71 | #### props.customClasses
|
72 |
|
73 | Type: `Object`
|
74 | Allowed Keys: `input`, `results`, `listItem`, `listAnchor`, `hover`
|
75 |
|
76 | An object containing custom class names for child elements. Useful for
|
77 | integrating with 3rd party UI kits.
|
78 |
|
79 | #### props.placeholder
|
80 |
|
81 | Type: `String`
|
82 |
|
83 | Placeholder text for the typeahead input.
|
84 |
|
85 | #### props.inputProps
|
86 |
|
87 | Type: `Object`
|
88 |
|
89 | Props to pass directly to the `<input>` element.
|
90 |
|
91 | #### props.onKeyDown
|
92 |
|
93 | Type: `Function`
|
94 |
|
95 | Event handler for the `keyDown` event on the typeahead input.
|
96 |
|
97 | #### props.onOptionSelected
|
98 |
|
99 | Type: `Function`
|
100 |
|
101 | Event handler triggered whenever a user picks an option.
|
102 |
|
103 | #### props.filterOption
|
104 |
|
105 | Type: `Function`
|
106 |
|
107 | A function to filter the provided `options` based on the current input value. For each option, receives `(inputValue, option)`. If not supplied, defaults to [fuzzy string matching](https://github.com/mattyork/fuzzy).
|
108 |
|
109 | ---
|
110 |
|
111 | ### Tokenizer(props)
|
112 |
|
113 | Type: React Component
|
114 |
|
115 | Typeahead component that allows for multiple options to be selected.
|
116 |
|
117 | #### props.options
|
118 |
|
119 | Type: `Array`
|
120 | Default: []
|
121 |
|
122 | An array supplied to the filter function.
|
123 |
|
124 | #### props.maxVisible
|
125 |
|
126 | Type: `Number`
|
127 |
|
128 | Limit the number of options rendered in the results list.
|
129 |
|
130 | #### props.name
|
131 |
|
132 | Type: `String`
|
133 |
|
134 | The name for HTML forms to be used for submitting the tokens' values array.
|
135 |
|
136 | #### props.customClasses
|
137 |
|
138 | Type: `Object`
|
139 | Allowed Keys: `input`, `results`, `listItem`, `listAnchor`, `typeahead`
|
140 |
|
141 | An object containing custom class names for child elements. Useful for
|
142 | integrating with 3rd party UI kits.
|
143 |
|
144 | #### props.placeholder
|
145 |
|
146 | Type: `String`
|
147 |
|
148 | Placeholder text for the typeahead input.
|
149 |
|
150 | #### props.inputProps
|
151 |
|
152 | Type: `Object`
|
153 |
|
154 | Props to pass directly to the `<input>` element.
|
155 |
|
156 | #### props.defaultSelected
|
157 |
|
158 | Type: `Array`
|
159 |
|
160 | A set of values of tokens to be loaded on first render.
|
161 |
|
162 | #### props.onTokenRemove
|
163 |
|
164 | Type: `Function`
|
165 |
|
166 | Event handler triggered whenever a token is removed.
|
167 |
|
168 | #### props.onTokenAdd
|
169 |
|
170 | Type: `Function`
|
171 |
|
172 | Event handler triggered whenever a token is removed.
|
173 |
|
174 | #### props.filterOption
|
175 |
|
176 | Type: `Function`
|
177 |
|
178 | A function to filter the provided `options` based on the current input value. For each option, receives `(inputValue, option)`. If not supplied, defaults to [fuzzy string matching](https://github.com/mattyork/fuzzy).
|
179 |
|
180 |
|
181 | ## Developing
|
182 |
|
183 | ### Setting Up
|
184 |
|
185 | You will need `npm` to develop on react-typeahead. [Installing npm][4].
|
186 |
|
187 | Once that's done, to get started, run `npm install` in your checkout directory.
|
188 | This will install all the local development dependences, such as `gulp` and `mocha`
|
189 |
|
190 | ### Testing
|
191 |
|
192 | react-typeahead uses mocha for unit tests and gulp for running them. Large changes should
|
193 | include unittests.
|
194 |
|
195 | After updating or creating new tests, run `npm run-script build-test` to regenerate the
|
196 | test package.
|
197 |
|
198 | Once that's done, running the tests is easy with `gulp`:
|
199 |
|
200 | ```
|
201 | > gulp test
|
202 | [00:17:25] Using gulpfile ~/src/react-typeahead/gulpfile.js
|
203 | [00:17:25] Starting 'test'...
|
204 |
|
205 |
|
206 | ․․․․․․․․․․․․․․․
|
207 |
|
208 | 15 passing (43ms)
|
209 |
|
210 | [00:17:25] Finished 'test' after 448 ms
|
211 | [00:17:25] Starting 'default'...
|
212 | [00:17:25] Finished 'default' after 6.23 μs
|
213 | ```
|
214 |
|
215 | ### Contributing
|
216 |
|
217 | Basically, fork the repository and send a pull request. It can be difficult to review these, so
|
218 | here are some general rules to follow for getting your PR accepted more quickly:
|
219 |
|
220 | - Break your changes into smaller, easy to understand commits.
|
221 | - Send separate PRs for each commit when possible.
|
222 | - Feel free to rebase, merge, and rewrite commits to make them more readible.
|
223 | - Add comments explaining anything that's not painfully obvious.
|
224 | - Add unittests for your change if possible.
|