1 | # react-typeahead
|
2 |
|
3 | > A typeahead/autocomplete component for React
|
4 |
|
5 | react-typeahead is a javascript library that provides a react-based
|
6 | typeahead, or autocomplete text entry, as well as a "typeahead tokenizer",
|
7 | a typeahead that allows you to select multiple results.
|
8 |
|
9 | ## Usage
|
10 |
|
11 | For a typeahead input:
|
12 |
|
13 | ```javascript
|
14 | var Typeahead = require('react-typeahead').Typeahead;
|
15 | React.render(
|
16 | <Typeahead
|
17 | options={['John', 'Paul', 'George', 'Ringo']}
|
18 | maxVisible={2}
|
19 | />
|
20 | );
|
21 | ```
|
22 |
|
23 | For a tokenizer typeahead input:
|
24 |
|
25 | ```javascript
|
26 | var Tokenizer = require('react-typeahead').Tokenizer;
|
27 | React.render(
|
28 | <Tokenizer
|
29 | options={['John', 'Paul', 'George', 'Ringo']}
|
30 | onTokenAdd={function(token) {
|
31 | console.log('token added: ', token);
|
32 | }}
|
33 | />
|
34 | );
|
35 | ```
|
36 |
|
37 | ## Examples
|
38 |
|
39 | * [Basic Typeahead with Topcoat][1]
|
40 | * [Typeahead Tokenizer with Topcoat][2]
|
41 | * [Typeahead Tokenizer with simple styling][3]
|
42 |
|
43 | 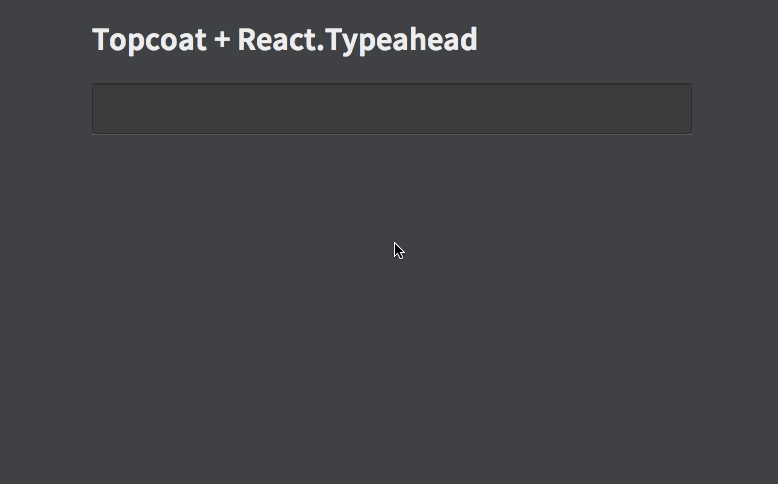
|
44 |
|
45 | [1]: http://wookiehangover.github.com/react-typeahead/examples/typeahead-topcoat.html
|
46 | [2]: http://wookiehangover.github.com/react-typeahead/examples/tokenizer-topcoat.html
|
47 | [3]: http://wookiehangover.github.com/react-typeahead/examples/TypeaheadTokenizer-simple.html
|
48 | [4]: http://blog.npmjs.org/post/85484771375/how-to-install-npm
|
49 |
|
50 | ## API
|
51 |
|
52 | ### Typeahead(props)
|
53 |
|
54 | Type: React Component
|
55 |
|
56 | Basic typeahead input and results list.
|
57 |
|
58 | #### props.options
|
59 |
|
60 | Type: `Array`
|
61 | Default: []
|
62 |
|
63 | An array supplied to the filtering function. Can be a list of strings or a list of arbitrary objects. In the latter case, `filterOption` and `displayOption` should be provided.
|
64 |
|
65 | #### props.defaultValue
|
66 |
|
67 | Type: `String`
|
68 |
|
69 | A default value used when the component has no value. If it matches any options a option list will show.
|
70 |
|
71 | #### props.value
|
72 |
|
73 | Type: `String`
|
74 |
|
75 | Specify a value for the text input.
|
76 |
|
77 | #### props.maxVisible
|
78 |
|
79 | Type: `Number`
|
80 |
|
81 | Limit the number of options rendered in the results list.
|
82 |
|
83 | #### props.customClasses
|
84 |
|
85 | Type: `Object`
|
86 | Allowed Keys: `input`, `results`, `listItem`, `listAnchor`, `hover`
|
87 |
|
88 | An object containing custom class names for child elements. Useful for
|
89 | integrating with 3rd party UI kits.
|
90 |
|
91 | #### props.placeholder
|
92 |
|
93 | Type: `String`
|
94 |
|
95 | Placeholder text for the typeahead input.
|
96 |
|
97 | #### props.textarea
|
98 |
|
99 | Type: `Boolean`
|
100 |
|
101 | Set to `true` to use a `<textarea>` element rather than an `<input>` element
|
102 |
|
103 | #### props.inputProps
|
104 |
|
105 | Type: `Object`
|
106 |
|
107 | Props to pass directly to the `<input>` element.
|
108 |
|
109 | #### props.onKeyDown
|
110 |
|
111 | Type: `Function`
|
112 |
|
113 | Event handler for the `keyDown` event on the typeahead input.
|
114 |
|
115 | #### props.onKeyUp
|
116 |
|
117 | Type: `Function`
|
118 |
|
119 | Event handler for the `keyUp` event on the typeahead input.
|
120 |
|
121 | #### props.onBlur
|
122 |
|
123 | Type: `Function`
|
124 |
|
125 | Event handler for the `blur` event on the typeahead input.
|
126 |
|
127 | #### props.onFocus
|
128 |
|
129 | Type: `Function`
|
130 |
|
131 | Event handler for the `focus` event on the typeahead input.
|
132 |
|
133 | #### props.onOptionSelected
|
134 |
|
135 | Type: `Function`
|
136 |
|
137 | Event handler triggered whenever a user picks an option.
|
138 |
|
139 | #### props.filterOption
|
140 |
|
141 | Type: `String` or `Function`
|
142 |
|
143 | A function to filter the provided `options` based on the current input value. For each option, receives `(inputValue, option)`. If not supplied, defaults to [fuzzy string matching](https://github.com/mattyork/fuzzy).
|
144 |
|
145 | If provided as a string, it will interpret it as a field name and fuzzy filter on that field of each option object.
|
146 |
|
147 | #### props.displayOption
|
148 |
|
149 | Type: `String` or `Function`
|
150 |
|
151 | A function to map an option onto a string for display in the list. Receives `(option, index)` where index is relative to the results list, not all the options. Must return a string.
|
152 |
|
153 | If provided as a string, it will interpret it as a field name and use that field from each option object.
|
154 |
|
155 | #### props.formInputOption
|
156 |
|
157 | Type: `String` or `Function`
|
158 |
|
159 | A function to map an option onto a string to include in HTML forms (see `props.name`). Receives `(option)` as arguments. Must return a string.
|
160 |
|
161 | If specified as a string, it will interpret it as a field name and use that field from each option object.
|
162 |
|
163 | If not specified, it will fall back onto the semantics described in `props.displayOption`.
|
164 |
|
165 | This option is ignored if you don't specify the `name` prop. It is required if you both specify the `name` prop and are using non-string options. It is optional otherwise.
|
166 |
|
167 | ### Typeahead ([Exposed Component Functions][reactecf])
|
168 |
|
169 | #### typeahead.focus
|
170 |
|
171 | Focuses the typeahead input.
|
172 |
|
173 | ---
|
174 |
|
175 | ### Tokenizer(props)
|
176 |
|
177 | Type: React Component
|
178 |
|
179 | Typeahead component that allows for multiple options to be selected.
|
180 |
|
181 | #### props.options
|
182 |
|
183 | Type: `Array`
|
184 | Default: []
|
185 |
|
186 | An array supplied to the filter function.
|
187 |
|
188 | #### props.maxVisible
|
189 |
|
190 | Type: `Number`
|
191 |
|
192 | Limit the number of options rendered in the results list.
|
193 |
|
194 | #### props.name
|
195 |
|
196 | Type: `String`
|
197 |
|
198 | The name for HTML forms to be used for submitting the tokens' values array.
|
199 |
|
200 | #### props.customClasses
|
201 |
|
202 | Type: `Object`
|
203 | Allowed Keys: `input`, `results`, `listItem`, `listAnchor`, `typeahead`
|
204 |
|
205 | An object containing custom class names for child elements. Useful for
|
206 | integrating with 3rd party UI kits.
|
207 |
|
208 | #### props.placeholder
|
209 |
|
210 | Type: `String`
|
211 |
|
212 | Placeholder text for the typeahead input.
|
213 |
|
214 | #### props.inputProps
|
215 |
|
216 | Type: `Object`
|
217 |
|
218 | Props to pass directly to the `<input>` element.
|
219 |
|
220 | #### props.onKeyDown
|
221 |
|
222 | Type: `Function`
|
223 |
|
224 | Event handler for the `keyDown` event on the typeahead input.
|
225 |
|
226 | #### props.onKeyUp
|
227 |
|
228 | Type: `Function`
|
229 |
|
230 | Event handler for the `keyUp` event on the typeahead input.
|
231 |
|
232 | #### props.onBlur
|
233 |
|
234 | Type: `Function`
|
235 |
|
236 | Event handler for the `blur` event on the typeahead input.
|
237 |
|
238 | #### props.onFocus
|
239 |
|
240 | Type: `Function`
|
241 |
|
242 | Event handler for the `focus` event on the typeahead input.
|
243 |
|
244 | #### props.defaultSelected
|
245 |
|
246 | Type: `Array`
|
247 |
|
248 | A set of values of tokens to be loaded on first render.
|
249 |
|
250 | #### props.onTokenRemove
|
251 |
|
252 | Type: `Function`
|
253 | Params: `(removedToken)`
|
254 |
|
255 | Event handler triggered whenever a token is removed.
|
256 |
|
257 | #### props.onTokenAdd
|
258 |
|
259 | Type: `Function`
|
260 | Params: `(addedToken)`
|
261 |
|
262 | Event handler triggered whenever a token is removed.
|
263 |
|
264 | #### props.displayOption
|
265 |
|
266 | Type: `String` or `Function`
|
267 |
|
268 | A function to map an option onto a string for display in the list. Receives `(option, index)` where index is relative to the results list, not all the options. Must return a string.
|
269 |
|
270 | If provided as a string, it will interpret it as a field name and use that field from each option object.
|
271 |
|
272 | #### props.filterOption
|
273 |
|
274 | Type: `Function`
|
275 |
|
276 | A function to filter the provided `options` based on the current input value. For each option, receives `(inputValue, option)`. If not supplied, defaults to [fuzzy string matching](https://github.com/mattyork/fuzzy).
|
277 |
|
278 | ### Tokenizer ([Exposed Component Functions][reactecf])
|
279 |
|
280 | #### tokenizer.focus
|
281 |
|
282 | Focuses the tokenizer input.
|
283 |
|
284 | #### tokenizer.getSelectedTokens
|
285 |
|
286 | Type: `Function`
|
287 |
|
288 | A function to return the currently selected tokens.
|
289 |
|
290 | ## Developing
|
291 |
|
292 | ### Setting Up
|
293 |
|
294 | You will need `npm` to develop on react-typeahead. [Installing npm][4].
|
295 |
|
296 | Once that's done, to get started, run `npm install` in your checkout directory.
|
297 | This will install all the local development dependences, such as `gulp` and `mocha`
|
298 |
|
299 | ### Testing
|
300 |
|
301 | react-typeahead uses mocha for unit tests and gulp for running them. Large changes should
|
302 | include unittests.
|
303 |
|
304 | After updating or creating new tests, run `npm run-script build-test` to regenerate the
|
305 | test package.
|
306 |
|
307 | Once that's done, running the tests is easy with `gulp`:
|
308 |
|
309 | ```
|
310 | > gulp test
|
311 | [00:17:25] Using gulpfile ~/src/react-typeahead/gulpfile.js
|
312 | [00:17:25] Starting 'test'...
|
313 |
|
314 |
|
315 | ․․․․․․․․․․․․․․․
|
316 |
|
317 | 15 passing (43ms)
|
318 |
|
319 | [00:17:25] Finished 'test' after 448 ms
|
320 | [00:17:25] Starting 'default'...
|
321 | [00:17:25] Finished 'default' after 6.23 μs
|
322 | ```
|
323 |
|
324 | ### Contributing
|
325 |
|
326 | Basically, fork the repository and send a pull request. It can be difficult to review these, so
|
327 | here are some general rules to follow for getting your PR accepted more quickly:
|
328 |
|
329 | - All new properties and exposed component function should be documented in the README.md
|
330 | - Break your changes into smaller, easy to understand commits.
|
331 | - Send separate PRs for each commit when possible.
|
332 | - Feel free to rebase, merge, and rewrite commits to make them more readible.
|
333 | - Add comments explaining anything that's not painfully obvious.
|
334 | - Add unittests for your change if possible.
|
335 |
|
336 | [reactecf]: https://facebook.github.io/react/tips/expose-component-functions.html
|