1 | # Short Unique ID (UUID) Generating Library
|
2 | 
|
3 | [](https://npm.runkit.com/short-unique-id)
|
4 | [](https://npmjs.com/package/short-unique-id)
|
5 | [](https://www.jsdelivr.com/package/npm/short-unique-id)
|
6 |
|
7 |
|
8 | [](#contributors)
|
9 |
|
10 |
|
11 | Generate random or sequential UUID of any length.
|
12 |
|
13 | This project is open to updates by its users, I ensure that PRs are relevant to the community.
|
14 | In other words, if you find a bug or want a new feature, please help us by becoming one of the
|
15 | [contributors](#contributors-) ✌️ ! See the [contributing section](#contributing).
|
16 |
|
17 | ## Like this module? ❤
|
18 |
|
19 | Please consider:
|
20 |
|
21 | - [Buying me a coffee](https://www.buymeacoffee.com/jeanlescure) ☕
|
22 | - Supporting me on [Patreon](https://www.patreon.com/jeanlescure) 🏆
|
23 | - Starring this repo on [Github](https://github.com/jeanlescure/short-unique-id) 🌟
|
24 |
|
25 | ## 📣 v4 Notice
|
26 |
|
27 | ### BREAKING CHANGES
|
28 |
|
29 | This project has been completely refactored from v3 to be less "Deno centric" and more good 'ol Typescript.
|
30 |
|
31 | We tried avoiding breaking changes, and generally succeded.
|
32 |
|
33 | With that said, for Node.js require use-cases:
|
34 |
|
35 | - The `dist` js files are now generated as UMD, named as `ShortUniqueId`, which means that...
|
36 |
|
37 | ```js
|
38 | // ...if you get the error "TypeError: ShortUniqueId is not a constructor" then this
|
39 | const { default: ShortUniqueId } = require('short-unique-id');
|
40 |
|
41 | // must be refactored to this
|
42 | const ShortUniqueId = require('short-unique-id');
|
43 | ```
|
44 |
|
45 | - The `lib` directory is now completely removed
|
46 |
|
47 | Also, the following changes might generate errors in some edge-cases:
|
48 |
|
49 | - The `short_uuid` Deno submodule has been completely removed from this repo and now all the logic lives in `src/index.ts`
|
50 | - Typings are no longer under the `typings` directory but are now under the `dist` directory
|
51 | - A sourcemap is now included along with the `dist` files
|
52 |
|
53 | ### New Features 🥳
|
54 |
|
55 | The ability to generate UUIDs that contain a timestamp which can be extracted:
|
56 |
|
57 | ```js
|
58 | const uid = new ShortUniqueId();
|
59 |
|
60 | const uidWithTimestamp = uid.stamp(32);
|
61 | console.log(uidWithTimestamp);
|
62 | // GDa608f973aRCHLXQYPTbKDbjDeVsSb3
|
63 |
|
64 | console.log(uid.parseStamp(uidWithTimestamp));
|
65 | // 2021-05-03T06:24:58.000Z
|
66 | ```
|
67 |
|
68 | Default dictionaries (generated on the spot to reduce memory footprint and
|
69 | avoid dictionary injection vulnerabilities):
|
70 |
|
71 | - number
|
72 | - alpha
|
73 | - alpha_lower
|
74 | - alpha_upper
|
75 | - **alphanum** _(default when no dictionary is provided to `new ShortUniqueId()`)_
|
76 | - alphanum_lower
|
77 | - alphanum_upper
|
78 | - hex
|
79 |
|
80 | ```js
|
81 | // instantiate using one of the default dictionary strings
|
82 | const uid = new ShortUniqueId({
|
83 | dictionary: 'hex', // the default
|
84 | });
|
85 |
|
86 | console.log(uid.dict.join());
|
87 | // 0,1,2,3,4,5,6,7,8,9,a,b,c,d,e,f
|
88 |
|
89 | // or change the dictionary after instantiation
|
90 | uid.setDictionary('alpha_upper');
|
91 |
|
92 | console.log(uid.dict.join());
|
93 | // A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z
|
94 | ```
|
95 |
|
96 | ### Use in CLI
|
97 |
|
98 | ```sh
|
99 | $ npm install -g short-unique-id
|
100 |
|
101 | $ short-unique-id -h
|
102 |
|
103 | # Usage:
|
104 | # node short-unique-id [OPTION]
|
105 |
|
106 | # Options:
|
107 | # -l, --length=ARG character length of the uid to generate.
|
108 | # -s, --stamp include timestamp in uid (must be used with --length (-l) of 10 or more).
|
109 | # -p, --parse=ARG extract timestamp from stamped uid (ARG).
|
110 | # -d, --dictionaryJson=ARG json file with dictionary array.
|
111 | # -h, --help display this help
|
112 | ```
|
113 |
|
114 | ### Use as module
|
115 |
|
116 | Add to your project:
|
117 |
|
118 | ```js
|
119 | // Deno (web module) Import
|
120 | import ShortUniqueId from 'https://cdn.jsdelivr.net/npm/short-unique-id@latest/src/index.ts';
|
121 |
|
122 | // ES6 / TypeScript Import
|
123 | import ShortUniqueId from 'short-unique-id';
|
124 |
|
125 | // Node.js require
|
126 | const ShortUniqueId = require('short-unique-id');
|
127 | ```
|
128 |
|
129 | Instantiate and use:
|
130 |
|
131 | ```js
|
132 | //Instantiate
|
133 | const uid = new ShortUniqueId();
|
134 |
|
135 | // Random UUID
|
136 | console.log(uid());
|
137 |
|
138 | // Sequential UUID
|
139 | console.log(uid.seq());
|
140 | ```
|
141 |
|
142 | ### Use in browser
|
143 |
|
144 | ```html
|
145 | <!-- Add source (minified 7.2K) -->
|
146 | <script src="https://cdn.jsdelivr.net/npm/short-unique-id@latest/dist/short-unique-id.min.js"></script>
|
147 |
|
148 | <!-- Usage -->
|
149 | <script>
|
150 | // Instantiate
|
151 | var uid = new ShortUniqueId();
|
152 |
|
153 | // Random UUID
|
154 | document.write(uid());
|
155 |
|
156 | // Sequential UUID
|
157 | document.write(uid.seq());
|
158 | </script>
|
159 | ```
|
160 |
|
161 | ### Options
|
162 |
|
163 | Options can be passed when instantiating `uid`:
|
164 |
|
165 | ```js
|
166 | const options = { ... };
|
167 |
|
168 | const uid = new ShortUniqueId(options);
|
169 | ```
|
170 |
|
171 | For more information take a look at the [docs](https://shortunique.id/interfaces/shortuniqueidoptions.html).
|
172 |
|
173 | ## Available for
|
174 |
|
175 | - [Node.js (npm)](https://www.npmjs.com/package/short-unique-id)
|
176 | - [Deno](https://www.jsdelivr.com/package/npm/short-unique-id?path=src)
|
177 | - [Browsers](https://www.jsdelivr.com/package/npm/short-unique-id?path=dist)
|
178 |
|
179 | ## Documentation with Online Short UUID Generator
|
180 |
|
181 | You can find the docs and online generator at:
|
182 |
|
183 | <a target="_blank" href="https://shortunique.id">https://shortunique.id</a>
|
184 |
|
185 | ## What is the probability of generating the same id again?
|
186 |
|
187 | This largely depends on the given dictionary and the selected UUID length.
|
188 |
|
189 | Out of the box this library provides a shuffled dictionary of digits from
|
190 | 0 to 9, as well as the alphabet from a to z both in UPPER and lower case,
|
191 | with a default UUID length of 6. That gives you a total of 56,800,235,584
|
192 | possible UUIDs.
|
193 |
|
194 | So, given the previous values, the probability of generating a duplicate
|
195 | in 1,000,000 rounds is ~0.00000002, or about 1 in 50,000,000.
|
196 |
|
197 | If you change the dictionary and/or the UUID length then we have provided
|
198 | the function `collisionProbability()` function to calculate the probability
|
199 | of hitting a duplicate in a given number of rounds (a collision) and the
|
200 | function `uniqueness()` which provides a score (from 0 to 1) to rate the
|
201 | "quality" of the combination of given dictionary and UUID length (the closer
|
202 | to 1, higher the uniqueness and thus better the quality).
|
203 |
|
204 | To find out more about the math behind these functions please refer to the
|
205 | <a target="_blank" href="https://shortunique.id/classes/default.html#collisionprobability">API Reference</a>.
|
206 |
|
207 | ## Acknowledgement and platform support
|
208 |
|
209 | This repo and npm package started as a straight up manual transpilation to ES6 of the [short-uid](https://github.com/serendipious/nodejs-short-uid) npm package by [Ankit Kuwadekar](https://github.com/serendipious/).
|
210 |
|
211 | 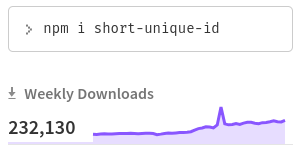
|
212 | 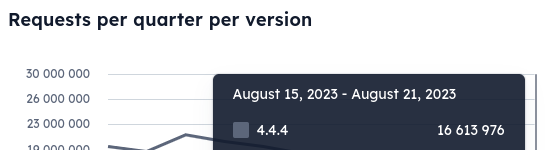
|
213 |
|
214 | Since this package is now reporting 12k+ npm weekly downloads and 100k+ weekly cdn hits,
|
215 | we've gone ahead and re-written the whole of it in TypeScript and made sure to package
|
216 | dist modules compatible with Deno, Node.js and all major Browsers.
|
217 |
|
218 | ## Development
|
219 |
|
220 | Clone this repo:
|
221 |
|
222 | ```sh
|
223 | # SSH
|
224 | git clone git@github.com:jeanlescure/short-unique-id.git
|
225 |
|
226 | # HTTPS
|
227 | git clone https://github.com/jeanlescure/short-unique-id.git
|
228 | ```
|
229 |
|
230 | Tests run using:
|
231 |
|
232 | ```
|
233 | yarn test
|
234 | ```
|
235 |
|
236 | ## Build
|
237 |
|
238 | In order to publish the latest changes you must build the distribution files:
|
239 |
|
240 | ```
|
241 | yarn build
|
242 | ```
|
243 |
|
244 | Then commit all changes and run the release script:
|
245 |
|
246 | ```
|
247 | yarn release
|
248 | ```
|
249 |
|
250 | ## Contributing
|
251 |
|
252 | Yes, thank you! This plugin is community-driven, most of its features are from different authors.
|
253 | Please update the docs and tests and add your name to the `package.json` file.
|
254 |
|
255 | ## Contributors ✨
|
256 |
|
257 | Thanks goes to these wonderful people ([emoji key](https://allcontributors.org/docs/en/emoji-key)):
|
258 |
|
259 |
|
260 |
|
261 |
|
262 | <table>
|
263 | <tr>
|
264 | <td align="center"><a href="https://github.com/serendipious"><img src="https://shortunique.id/assets/contributors/serendipious.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short-unique-id/commits?author=serendipious" title="Code">💻</a></td></tr></tbody></table></td>
|
265 | <td align="center"><a href="https://jeanlescure.cr"><img src="https://shortunique.id/assets/contributors/jeanlescure.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="#maintenance-jeanlescure" title="Maintenance">🚧</a> <a href="https://github.com/jeanlescure/short-unique-id/commits?author=jeanlescure" title="Code">💻</a> <a href="#content-jeanlescure" title="Content">🖋</a> <a href="https://github.com/jeanlescure/short-unique-id/commits?author=jeanlescure" title="Documentation">📖</a> <a href="https://github.com/jeanlescure/short-unique-id/commits?author=jeanlescure" title="Tests">⚠️</a></td></tr></tbody></table></td>
|
266 | <td align="center"><a href="https://dianalu.design"><img src="https://shortunique.id/assets/contributors/dilescure.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short_uuid/commits?author=DiLescure" title="Code">💻</a></td></tr></tbody></table></td>
|
267 | <td align="center"><a href="https://github.com/EmerLM"><img src="https://shortunique.id/assets/contributors/emerlm.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short_uuid/commits?author=EmerLM" title="Code">💻</a></td></tr></tbody></table></td>
|
268 | </tr>
|
269 | <tr>
|
270 | <td align="center"><a href="https://github.com/angelnath26"><img src="https://shortunique.id/assets/contributors/angelnath26.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short_uuid/commits?author=angelnath26" title="Code">💻</a> <a href="https://github.com/jeanlescure/short_uuid/pulls?q=is%3Apr+reviewed-by%3Aangelnath26" title="Reviewed Pull Requests">👀</a></td></tr></tbody></table></td>
|
271 | <td align="center"><a href="https://twitter.com/jeffturcotte"><img src="https://shortunique.id/assets/contributors/jeffturcotte.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short-unique-id/commits?author=jeffturcotte" title="Code">💻</a></td></tr></tbody></table></td>
|
272 | <td align="center"><a href="https://github.com/neversun"><img src="https://shortunique.id/assets/contributors/neversun.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short-unique-id/commits?author=neversun" title="Code">💻</a></td></tr></tbody></table></td>
|
273 | <td align="center"><a href="https://github.com/ekelvin"><img src="https://shortunique.id/assets/contributors/ekelvin.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short-unique-id/issues/19" title="Ideas, Planning, & Feedback">🤔</a></td></tr></tbody></table></td>
|
274 | </tr>
|
275 | <tr>
|
276 | <td align="center"><a href="https://github.com/anthony-arnold"><img src="https://shortunique.id/assets/contributors/anthony-arnold.svg" /></a><table><tbody><tr><td width="150" align="center"><a href="https://github.com/jeanlescure/short-unique-id/issues/35" title="Security">🛡️</a></td></tr></tbody></table></td>
|
277 | </tr>
|
278 | </table>
|
279 |
|
280 |
|
281 |
|
282 |
|
283 |
|
284 | ## License
|
285 |
|
286 | Copyright (c) 2018-2021 [Short Unique ID Contributors](https://github.com/jeanlescure/short-unique-id/#contributors-).<br/>
|
287 | Licensed under the [Apache License 2.0](https://www.apache.org/licenses/LICENSE-2.0).
|