1 | social-cms-backend
|
2 | ==================
|
3 |
|
4 | Express middleware to provide schema-less REST APIs for creating a social networking website primarily using angular.js. It comes with built-in authentication, authorization and notification features.
|
5 |
|
6 | Motivation
|
7 | ----------
|
8 |
|
9 | There exists several MVC framework libraries for node.js
|
10 | that are inspired by Rails. But they might be a bit outdated,
|
11 | when it comes to angular.js, client-side MVW framework.
|
12 | I would like to propose a maybe new style of web programming,
|
13 | which is the combination of a domain-specific REST API library
|
14 | (ready to use, no coding required) and client-side coding.
|
15 |
|
16 | This project is to provide such a library for a web site
|
17 | like SNS/Twitter/Facebook in a closed/private environment.
|
18 |
|
19 | How to install
|
20 | --------------
|
21 |
|
22 | $ npm install social-cms-backend
|
23 |
|
24 | How to use
|
25 | ----------
|
26 |
|
27 | var express = require('express');
|
28 | var SCB = require('social-cms-backend');
|
29 | var app = express();
|
30 | app.use(SCB.middleware({
|
31 | mongodb_url: 'mongodb://localhost:27017/socialcmsdb',
|
32 | passport_strategy: 'facebook',
|
33 | facebook_app_id: process.env.FACEBOOK_APP_ID,
|
34 | facebook_app_secret: process.env.FACEBOOK_APP_SECRET
|
35 | }));
|
36 | app.listen(3000);
|
37 |
|
38 | With socket.io 1.0 (upcoming):
|
39 |
|
40 | var http = require('http');
|
41 | var express = require('express');
|
42 | var socket_io = require('socket.io');
|
43 | var SCB = require('social-cms-backend');
|
44 | var app = express();
|
45 | var SCB_options = {
|
46 | mongodb_url: 'mongodb://localhost:27017/socialcmsdb',
|
47 | passport_strategy: 'facebook',
|
48 | facebook_app_id: process.env.FACEBOOK_APP_ID,
|
49 | facebook_app_secret: process.env.FACEBOOK_APP_SECRET
|
50 | };
|
51 | app.use(SCB.middleware(SCB_options));
|
52 | var server = http.createServer(app);
|
53 | var sio = socket_io(server);
|
54 | sio.use(SCB.socket_io(SCB_options));
|
55 | server.listen(3000);
|
56 |
|
57 | With HTTP DIGEST strategy:
|
58 |
|
59 | var SCB_options = {
|
60 | mongodb_url: 'mongodb://localhost:27017/socialcmsdb',
|
61 | passport_strategy: 'digest',
|
62 | auth_digest: {
|
63 | realm: 'my_realm'
|
64 | }
|
65 | };
|
66 |
|
67 | REST APIs
|
68 | ---------
|
69 |
|
70 | By default, there are 4 objects:
|
71 | * post
|
72 | * user
|
73 | * group
|
74 | * like
|
75 |
|
76 | The following is the example of the post object endpoints.
|
77 |
|
78 | POST /posts (body: a JSON w/o system preserved keys)
|
79 |
|
80 | GET /posts?query=... (query: MongoDB query parameter stringified)
|
81 |
|
82 | GET /posts/inbox
|
83 |
|
84 | GET /posts/count?query=...
|
85 |
|
86 | GET /posts/123
|
87 |
|
88 | PUT /posts/123 (body: MongoDB update object, using update operators)
|
89 |
|
90 | DELETE /posts/123
|
91 |
|
92 | A special endpoint:
|
93 |
|
94 | GET /users/myself
|
95 |
|
96 | Screencast
|
97 | ----------
|
98 |
|
99 | ### How to create a Twitter clone in 15 minutes
|
100 |
|
101 | Screencast preview (quadruple speed):
|
102 |
|
103 | 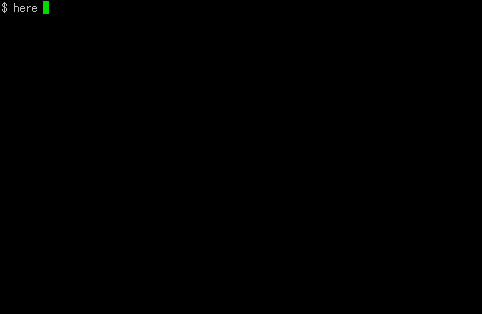
|
104 |
|
105 | <a href="http://dai-shi.github.io/social-cms-backend/ttyplay.html" target="_blank">Controllable screencast at normal speed</a>
|
106 |
|
107 | Notes:
|
108 |
|
109 | * There is a typo found after the recording.
|
110 | `/javascript/main.js -> /javascripts/main.js`
|
111 | * The resulting code is available
|
112 | [here](https://github.com/dai-shi/twitter-clone-sample/tree/20130804_recorded)
|
113 | * You can try the running web service of the code
|
114 | <a href="http://twitterclonesample-nodeangularapp.rhcloud.com/" target="_blank">here</a>
|
115 |
|
116 | TODOs
|
117 | -----
|
118 |
|
119 | * Notification: email
|