1 | [](https://travis-ci.org/googleapis/teeny-request)
|
2 |
|
3 | # teeny-request
|
4 |
|
5 | Like `request`, but much smaller - and with less options. Uses `node-fetch` under the hood.
|
6 | Pop it in where you would use `request`. Improves load and parse time of modules.
|
7 |
|
8 | ```js
|
9 | const request = require('teeny-request').teenyRequest;
|
10 |
|
11 | request({uri: 'http://ip.jsontest.com/'}, function (error, response, body) {
|
12 | console.log('error:', error); // Print the error if one occurred
|
13 | console.log('statusCode:', response && response.statusCode); // Print the response status code if a response was received
|
14 | console.log('body:', body); // Print the JSON.
|
15 | });
|
16 | ```
|
17 |
|
18 | For TypeScript, you can use `@types/request`.
|
19 |
|
20 | ```ts
|
21 | import {teenyRequest as request} from 'teeny-request';
|
22 | import r as * from 'request'; // Only for type declarations
|
23 |
|
24 | request({uri: 'http://ip.jsontest.com/'}, (error: any, response: r.Response, body: any) => {
|
25 | console.log('error:', error); // Print the error if one occurred
|
26 | console.log('statusCode:', response && response.statusCode); // Print the response status code if a response was received
|
27 | console.log('body:', body); // Print the JSON.
|
28 | });
|
29 | ```
|
30 |
|
31 |
|
32 |
|
33 | ## teenyRequest(options, callback)
|
34 |
|
35 | Options are limited to the following
|
36 |
|
37 | * uri
|
38 | * method, default GET
|
39 | * headers
|
40 | * json
|
41 | * qs
|
42 | * useQuerystring
|
43 | * timeout in ms
|
44 | * gzip
|
45 | * proxy
|
46 |
|
47 | ```ts
|
48 | request({uri:'http://service.com/upload', method:'POST', json: {key:'value'}}, function(err,httpResponse,body){ /* ... */ })
|
49 | ```
|
50 |
|
51 | The callback argument gets 3 arguments:
|
52 |
|
53 | * An error when applicable (usually from http.ClientRequest object)
|
54 | * An response object with statusCode, a statusMessage, and a body
|
55 | * The third is the response body (JSON object)
|
56 |
|
57 | ## defaults(options)
|
58 |
|
59 | Set default options for every `teenyRequest` call.
|
60 |
|
61 | ```ts
|
62 | let defaultRequest = teenyRequest.defaults({timeout: 60000});
|
63 | defaultRequest({uri: 'http://ip.jsontest.com/'}, function (error, response, body) {
|
64 | assert.ifError(error);
|
65 | assert.strictEqual(response.statusCode, 200);
|
66 | console.log(body.ip);
|
67 | assert.notEqual(body.ip, null);
|
68 |
|
69 | done();
|
70 | });
|
71 | ```
|
72 |
|
73 | ## Proxy environment variables
|
74 | If environment variables `HTTP_PROXY`, `HTTPS_PROXY`, or `NO_PROXY` are set, they are respected.
|
75 |
|
76 | ## Building with Webpack 4+
|
77 | Since 4.0.0, Webpack uses `javascript/esm` for `.mjs` files which handles ESM more strictly compared to `javascript/auto`. If you get the error `Can't import the named export 'PassThrough' from non EcmaScript module`, please add the following to your Webpack config:
|
78 |
|
79 | ```js
|
80 | {
|
81 | test: /\.mjs$/,
|
82 | type: 'javascript/auto',
|
83 | },
|
84 | ```
|
85 |
|
86 | ## Motivation
|
87 | `request` has a ton of options and features and is accordingly large. Requiering a module incurs load and parse time. For
|
88 | `request`, that is around 600ms.
|
89 |
|
90 | 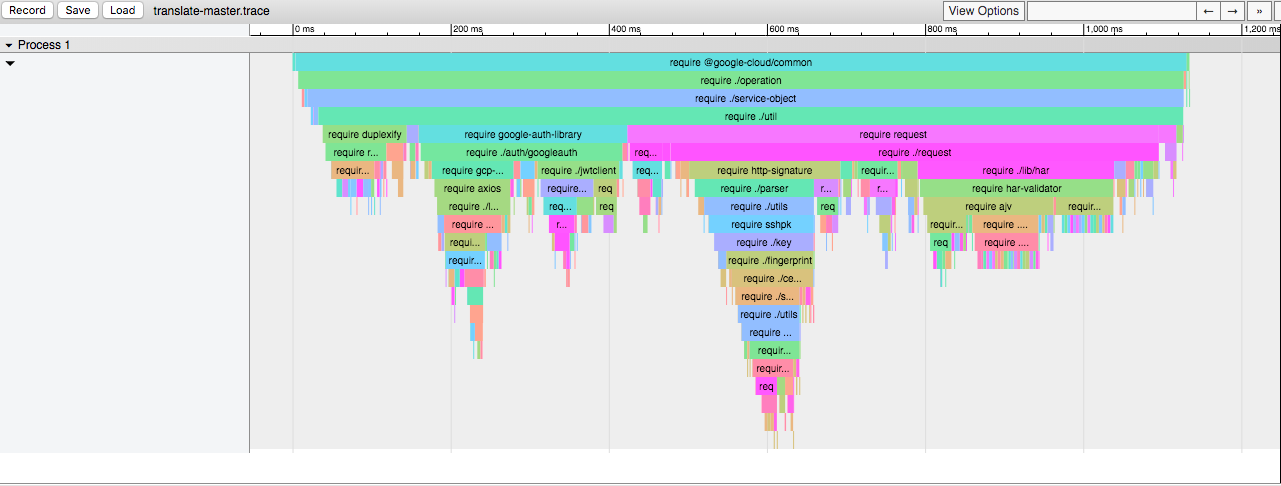
|
91 |
|
92 | `teeny-request` doesn't have any of the bells and whistles that `request` has, but is so much faster to load. If startup time is an issue and you don't need much beyong a basic GET and POST, you can use `teeny-request`.
|
93 |
|
94 | ## Thanks
|
95 | Special thanks to [billyjacobson](https://github.com/billyjacobson) for suggesting the name. Please report all bugs to them. Just kidding. Please open issues.
|