1 | # Twitter API v1.1 OAuth 1.0a 🔑
|
2 |
|
3 | [](https://www.npmjs.com/package/twitter-v1-oauth)
|
4 | [](https://github.com/MauricioRobayo/twitter-v1-oauth/actions/workflows/main.yml)
|
5 | [](https://codecov.io/gh/MauricioRobayo/twitter-v1-oauth)
|
6 | [](https://www.codefactor.io/repository/github/mauriciorobayo/twitter-v1-oauth)
|
7 |
|
8 | Simple and minimalist module to send OAuth 1.0a requests to the Twitter API v1.1.
|
9 |
|
10 | It returns an object with the request options necessary to make a request using your favorite tool.
|
11 |
|
12 | ```typescript
|
13 | import dotenv from "dotenv";
|
14 | import oAuthV1Request from "twitter-v1-oauth";
|
15 | import axios from "axios";
|
16 |
|
17 | dotenv.config();
|
18 |
|
19 | const oAuthOptions = {
|
20 | api_key: process.env.TWITTER_API_KEY || "",
|
21 | api_secret_key: process.env.TWITTER_API_SECRET_KEY || "",
|
22 | access_token: process.env.TWITTER_ACCESS_TOKEN || "",
|
23 | access_token_secret: process.env.TWITTER_ACCESS_TOKEN_SECRET || "",
|
24 | };
|
25 | const baseURL = "https://api.twitter.com/1.1/search/tweets.json";
|
26 | const method = "GET";
|
27 | const params = { q: "twitter bot" };
|
28 |
|
29 | const searchRequest = oAuthV1Request({
|
30 | oAuthOptions,
|
31 | method,
|
32 | baseURL,
|
33 | params,
|
34 | });
|
35 |
|
36 | axios
|
37 | .request(searchRequest)
|
38 | .then(({ data }) => console.log(data))
|
39 | .catch((err) => {
|
40 | if (err.response) {
|
41 | return console.log(err.response);
|
42 | }
|
43 | console.log(err);
|
44 | });
|
45 | ```
|
46 |
|
47 | No dependencies and super small: [](https://packagephobia.now.sh/result?p=twitter-v1-oauth).
|
48 |
|
49 | 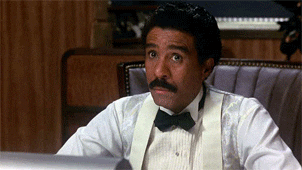
|
50 |
|
51 | ## Install
|
52 |
|
53 | ```shell
|
54 | npm install twitter-v1-oauth
|
55 | ```
|
56 |
|
57 | ## Usage
|
58 |
|
59 | Create an app and get your credentials, you will need:
|
60 |
|
61 | - API KEY
|
62 | - API SECRET KEY
|
63 | - ACCESS TOKEN
|
64 | - ACCESS TOKEN SECRET
|
65 |
|
66 | Use your preferred library to send the request using the documented endpoints and parameters for the [twitter v1 API](https://developer.twitter.com/en/docs/basics/getting-started).
|
67 |
|
68 | #### CommonJS
|
69 |
|
70 | ```js
|
71 | const authRequest = require("twitter-v1-oauth").default;
|
72 | ```
|
73 |
|
74 | #### ES6 Modules
|
75 |
|
76 | ```js
|
77 | import oAuthV1Request from "twitter-v1-oauth";
|
78 | ```
|
79 |
|
80 | #### TypeScript
|
81 |
|
82 | Type definitions are included.
|
83 |
|
84 | ## Examples
|
85 |
|
86 | Check the [examples](./examples) directory for ideas on how to use it with [axios](https://github.com/axios/axios).
|
87 |
|
88 | The [index.test.ts](./src/index.test.ts) file should also provide a good idea on its usage.
|
89 |
|
90 | ## Twitter documentation
|
91 |
|
92 | - [Authentication](https://developer.twitter.com/en/docs/basics/authentication/overview/oauth)
|
93 | - [Authorizing a request](https://developer.twitter.com/en/docs/basics/authentication/guides/authorizing-a-request.html)
|
94 | - [Creating a signature](https://developer.twitter.com/en/docs/basics/authentication/guides/authorizing-a-request.html)
|
95 |
|
96 | ## License
|
97 |
|
98 | [MIT](./LICENSE)
|