1 | # uwt [](https://npmjs.org/package/uwt) [](https://npmjs.org/package/uwt) [](http://standardjs.com/) [](LICENSE)
|
2 |
|
3 | #### µWT (µWebTorrentTracker) is a simple, robust and lightweight WebTorrent tracker server implementation
|
4 | [](https://travis-ci.org/DiegoRBaquero/uWebTorrentTracker) [](https://www.bithound.io/github/DiegoRBaquero/uWebTorrentTracker)
|
5 |
|
6 |
|
7 | 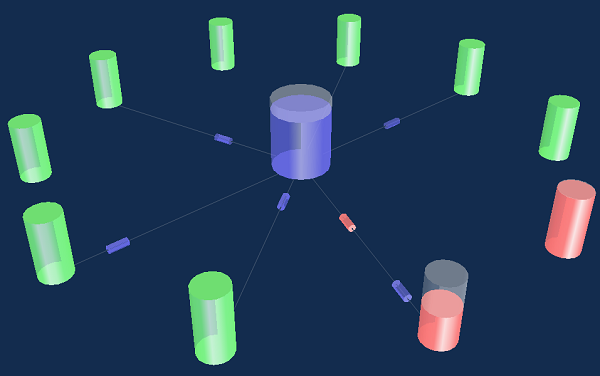
|
8 |
|
9 | Node.js implementation of a [BitTorrent tracker](https://wiki.theory.org/BitTorrentSpecification#Tracker_HTTP.2FHTTPS_Protocol) for WebTorrent clients.
|
10 |
|
11 | A **BitTorrent tracker** is a web service which responds to requests from BitTorrent
|
12 | clients. The requests include metrics from clients that help the tracker keep overall
|
13 | statistics about the torrent. The response includes a peer list that helps the client
|
14 | participate in the torrent swarm.
|
15 |
|
16 | This module is used by [βTorrent Tracker](https://tracker.btorrent.xyz), the first community operated [WebTorrent](http://webtorrent.io) tracker.
|
17 |
|
18 | ## Features
|
19 |
|
20 | - Fast & lightweight server implementation
|
21 | - Supports ipv4 & ipv6
|
22 | - Supports tracker "scrape" extension
|
23 | - Robust and well-tested
|
24 | - Comprehensive test suite (runs entirely offline, so it's reliable)
|
25 | - Tracker statistics available via web interface at `/stats` or JSON data at `/stats.json`
|
26 |
|
27 | ### Requires NodeJS 6+
|
28 |
|
29 | ## Install
|
30 |
|
31 | ```
|
32 | npm install uwt
|
33 | ```
|
34 |
|
35 | ## Usage
|
36 |
|
37 | To start a WebTorrent tracker server to track swarms of peers:
|
38 |
|
39 | ```js
|
40 | const Server = require('uwt')
|
41 |
|
42 | const server = new Server({
|
43 | stats: true, // enable web-based statistics? [default=true]
|
44 | filter: function (infoHash, params, cb) {
|
45 | // Blacklist/whitelist function for allowing/disallowing torrents. If this option is
|
46 | // omitted, all torrents are allowed. It is possible to interface with a database or
|
47 | // external system before deciding to allow/deny, because this function is async.
|
48 |
|
49 | // It is possible to block by peer id (whitelisting torrent clients) or by secret
|
50 | // key (private trackers). Full access to the original HTTP/UDP request parameters
|
51 | // are available in `params`.
|
52 |
|
53 | // This example only allows one torrent.
|
54 |
|
55 | const allowed = (infoHash === 'aaa67059ed6bd08362da625b3ae77f6f4a075aaa')
|
56 | if (allowed) {
|
57 | cb(null)
|
58 | } else {
|
59 | cb(new Error('disallowed torrent'))
|
60 | }
|
61 |
|
62 | // In addition to returning a boolean (`true` for allowed, `false` for disallowed),
|
63 | // you can return an `Error` object to disallow and provide a custom reason.
|
64 | }
|
65 | })
|
66 |
|
67 | // Internal websocket and http servers exposed as public properties.
|
68 | server.ws
|
69 | server.http
|
70 |
|
71 | server.on('error', function (err) {
|
72 | // fatal server error!
|
73 | console.log(err.message)
|
74 | })
|
75 |
|
76 | server.on('warning', function (err) {
|
77 | // client sent bad data. probably not a problem, just a buggy client.
|
78 | console.log(err.message)
|
79 | })
|
80 |
|
81 | server.on('listening', function () {
|
82 | // fired when server is listening
|
83 | console.log('listening on http port:' + server.http.address().port)
|
84 | })
|
85 |
|
86 | // start tracker server listening! Use 0 to listen on a random free port.
|
87 | server.listen(port, onlistening)
|
88 |
|
89 | // listen for individual tracker messages from peers:
|
90 |
|
91 | server.on('start', function (addr) {
|
92 | console.log('got start message from ' + addr)
|
93 | })
|
94 |
|
95 | server.on('complete', function (addr) {})
|
96 | server.on('update', function (addr) {})
|
97 | server.on('stop', function (addr) {})
|
98 |
|
99 | // get info hashes for all torrents in the tracker server
|
100 | Object.keys(server.torrents)
|
101 |
|
102 | // get the number of seeders for a particular torrent
|
103 | server.torrents[infoHash].complete
|
104 |
|
105 | // get the number of leechers for a particular torrent
|
106 | server.torrents[infoHash].incomplete
|
107 |
|
108 | // get the peers who are in a particular torrent swarm
|
109 | server.torrents[infoHash].peers
|
110 | ```
|
111 |
|
112 | ## CLI
|
113 |
|
114 | Easily start a tracker server:
|
115 |
|
116 | ```sh
|
117 | $ webtorrent-tracker
|
118 | Tracker: ws://localhost:8000
|
119 | Tracker stats: http://localhost:8000/stats
|
120 | ```
|
121 |
|
122 | Lots of options:
|
123 |
|
124 | ```sh
|
125 | $ webtorrent-tracker --help
|
126 | webtorrent-tracker - Start a webtorrent tracker server
|
127 |
|
128 | Usage:
|
129 | webtorrent-tracker [OPTIONS]
|
130 |
|
131 | Options:
|
132 | -p, --port [number] change the port [default: 8000]
|
133 | --trust-proxy trust 'x-forwarded-for' header from reverse proxy
|
134 | --interval client announce interval (ms) [default: 120000]
|
135 | --stats enable web-based statistics (default: true)
|
136 | -q, --quiet only show error output
|
137 | -s, --silent show no output
|
138 | -v, --version print the current version
|
139 |
|
140 | Please report bugs! https://github.com/DiegoRBaquero/uwt/issues
|
141 | ```
|
142 |
|
143 | ## License
|
144 |
|
145 | MIT
|
146 |
|
147 | Copyright (c) [Diego Rodríguez Baquero](https://diegorbaquero.com) (uwt)
|
148 |
|
149 | Copyright (c) [Feross Aboukhadijeh](http://feross.org) (bittorrent-tracker)
|