1 | <p align="center">
|
2 | <a href="https://vizzu-story.vizzuhq.com/0.7/">
|
3 | <img src="https://vizzu-story.vizzuhq.com/0.7/assets/vizzu-story.gif" alt="Vizzu-Story" />
|
4 | </a>
|
5 | <p align="center"><b>Vizzu-Story</b> - Build and present animated data stories</p>
|
6 | <p align="center">
|
7 | <a href="https://vizzu-story.vizzuhq.com/0.7/">Documentation</a>
|
8 | · <a href="https://vizzu-story.vizzuhq.com/0.7/examples/">Examples</a>
|
9 | · <a href="https://vizzu-story.vizzuhq.com/0.7/reference/">Code reference</a>
|
10 | · <a href="https://github.com/vizzuhq/vizzu-story-js/">Repository</a>
|
11 | · <a href="https://blog.vizzuhq.com">Blog</a>
|
12 | </p>
|
13 | </p>
|
14 |
|
15 | [](https://badge.fury.io/js/vizzu-story)
|
16 | [](https://packagephobia.com/result?p=vizzu-story)
|
17 |
|
18 | # Vizzu-Story
|
19 |
|
20 | ## About The Extension
|
21 |
|
22 | `Vizzu-Story` is an extension for the
|
23 | [Vizzu](https://github.com/vizzuhq/vizzu-lib) `JavaScript` library that allows
|
24 | users to create interactive presentations from the animated data visualizations
|
25 | built with `Vizzu`.
|
26 |
|
27 | The extension provides a `Web Component` that contains the presentation and adds
|
28 | controls for navigating between slides - predefined stages within the story.
|
29 |
|
30 | ## Installation
|
31 |
|
32 | Install via [npm](https://www.npmjs.com/package/vizzu-story):
|
33 |
|
34 | ```sh
|
35 | npm install vizzu-story
|
36 | ```
|
37 |
|
38 | Or use it from [CDN](https://www.jsdelivr.com/package/npm/vizzu-story):
|
39 |
|
40 | ```javascript
|
41 | import VizzuPlayer from 'https://cdn.jsdelivr.net/npm/vizzu-story@0.7/dist/vizzu-story.min.js';
|
42 | ```
|
43 |
|
44 | ## Usage
|
45 |
|
46 | 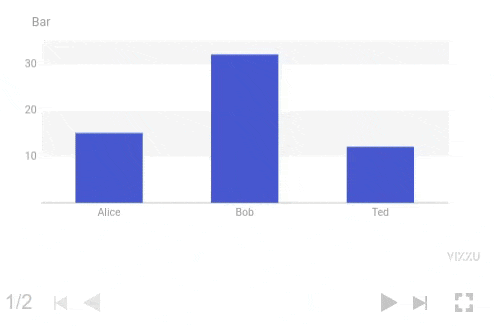
|
47 |
|
48 | Create a `vizzu-player` element that will contain the rendered story:
|
49 |
|
50 | ```
|
51 | <vizzu-player controller></vizzu-player>
|
52 | ```
|
53 |
|
54 | In a script module element import the extension from `CDN` or local install:
|
55 |
|
56 | ```
|
57 | <script type="module">
|
58 | import VizzuPlayer from
|
59 | 'https://cdn.jsdelivr.net/npm/vizzu-story@0.7/dist/vizzu-story.min.js';
|
60 | </script>
|
61 | ```
|
62 |
|
63 | Add the underlying data for the data story. You can use the same data definition
|
64 | formats as in the `Vizzu` library, but you must add the entire data set for the
|
65 | whole story in the initial step; you can not change this later. See
|
66 | [Data chapter](https://vizzu-story.vizzuhq.com/0.7/tutorial/data/) for more
|
67 | details on data formats.
|
68 |
|
69 | ```javascript
|
70 | const data = {
|
71 | series: [{
|
72 | name: 'Foo',
|
73 | values: ['Alice', 'Bob', 'Ted']
|
74 | }, {
|
75 | name: 'Bar',
|
76 | values: [15, 32, 12]
|
77 | }, {
|
78 | name: 'Baz',
|
79 | values: [5, 3, 2]
|
80 | }]
|
81 | };
|
82 | ```
|
83 |
|
84 | Create the data story by defining a sequence of slides. A slide can be a single
|
85 | chart corresponding to an [animate](https://lib.vizzuhq.com/0.9/tutorial/)
|
86 | call from `Vizzu`. Or a slide can be a sequence of animation calls, in which
|
87 | case all of these animations will be played until the last one in the sequence,
|
88 | allowing for more complex transitions between slides.
|
89 |
|
90 | ```javascript
|
91 | const slides = [{
|
92 | config: {
|
93 | x: 'Foo',
|
94 | y: 'Bar'
|
95 | }
|
96 | }, {
|
97 | config: {
|
98 | color: 'Foo',
|
99 | x: 'Baz',
|
100 | geometry: 'circle'
|
101 | }
|
102 | }];
|
103 | ```
|
104 |
|
105 | Navigation controls beneath the chart will navigate between the slides. You can
|
106 | use the `PgUp` and `PgDn` buttons, left and right arrows to navigate between
|
107 | slides, and the `Home` and `End` buttons to jump to the first or last slide.
|
108 |
|
109 | On each chart, you can define the chart configuration and style with the same
|
110 | objects as in `Vizzu`. However, you can not modify the underlying data between
|
111 | the slides, only the data filter used.
|
112 |
|
113 | ```typescript
|
114 | interface Chart {
|
115 | config?: Vizzu.Config.Chart;
|
116 | filter?: Vizzu.Data.FilterCallback | null;
|
117 | style?: Vizzu.Styles.Chart;
|
118 | animOptions?: Vizzu.Anim.Options;
|
119 | }
|
120 | ```
|
121 |
|
122 | Put the data and the slide list into the `story` descriptor object. Here you can
|
123 | also set the `story` `style` property to set the chart style used for the whole
|
124 | `story`.
|
125 |
|
126 | ```javascript
|
127 | const story = {
|
128 | data: data,
|
129 | slides: slides
|
130 | };
|
131 | ```
|
132 |
|
133 | Then set up the created element with the configuration object:
|
134 |
|
135 | ```javascript
|
136 | const vp = document.querySelector('vizzu-player');
|
137 | vp.slides = story;
|
138 | ```
|
139 |
|
140 | > [Check out a live example in JSFiddle!](https://jsfiddle.net/VizzuHQ/topcmuyf/3/)
|
141 |
|
142 | ## Documentation
|
143 |
|
144 | Visit our [Documentation site](https://vizzu-story.vizzuhq.com/0.7/) for more
|
145 | details and a step-by-step tutorial into `Vizzu-Story` or check out our
|
146 | [Example gallery](https://vizzu-story.vizzuhq.com/0.7/examples/).
|
147 |
|
148 | ## Contributing
|
149 |
|
150 | We welcome contributions to the project; visit our
|
151 | [Contributing guide](https://vizzu-story.vizzuhq.com/0.7/CONTRIBUTING/) for
|
152 | further info.
|
153 |
|
154 | ## Contact
|
155 |
|
156 | - Join our Slack:
|
157 | [vizzu-community.slack.com](https://join.slack.com/t/vizzu-community/shared_invite/zt-w2nqhq44-2CCWL4o7qn2Ns1EFSf9kEg)
|
158 | - Drop us a line at hello@vizzuhq.com
|
159 | - Follow us on Twitter:
|
160 | [https://twitter.com/VizzuHQ](https://twitter.com/VizzuHQ)
|
161 |
|
162 | ## License
|
163 |
|
164 | Copyright © 2022-2023 [Vizzu Inc.](https://vizzuhq.com)
|
165 |
|
166 | Released under the
|
167 | [Apache 2.0 License](https://vizzu-story.vizzuhq.com/0.7/LICENSE/).
|