1 | # 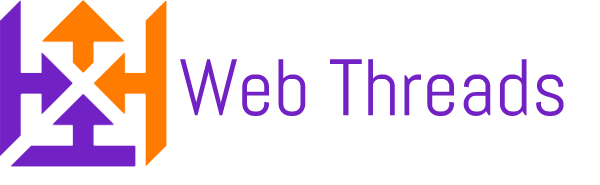
|
2 | [](https://travis-ci.org/kanekotic/web-threads)
|
3 | [](https://codecov.io/gh/kanekotic/web-threads)
|
4 | [](https://github.com/kanekotic/web-threads)
|
5 | [](https://github.com/kanekotic/web-threads/blob/master/LICENSE)
|
6 | [](https://GitHub.com/kanekotic/web-threads/graphs/commit-activity)
|
7 | [](https://www.paypal.me/kanekotic/)
|
8 |
|
9 | generic threads using web workers for the web
|
10 |
|
11 | ## Installation
|
12 |
|
13 | add it to your project using `npm install web-threads --save` or `yarn add web-threads`
|
14 |
|
15 | ## Usage
|
16 |
|
17 | ### Simple function with arguments
|
18 |
|
19 | ```js
|
20 | import { execute } from 'web-threads'
|
21 |
|
22 | let func = (value) => {
|
23 | return value * value
|
24 | }
|
25 | let params = {
|
26 | fn: func.toString(),
|
27 | args: [2]
|
28 | }
|
29 | execute(params)
|
30 | .then(console.log)
|
31 | .catch(console.error)
|
32 | ```
|
33 |
|
34 | ### Function with context
|
35 |
|
36 | ```js
|
37 | import { execute } from 'web-threads'
|
38 |
|
39 | function Func(value){
|
40 | this.value = value
|
41 | }
|
42 | Func.prototype.foo = function(){
|
43 | return this.value * this.value
|
44 | };
|
45 | var instance = new Func(2)
|
46 | let params = {
|
47 | fn: instance.foo,
|
48 | context: instance
|
49 | }
|
50 | execute(params)
|
51 | .then(console.log)
|
52 | .catch(console.error)
|
53 | ```
|
54 |
|
55 | ### Function with context and arguments
|
56 |
|
57 | ```js
|
58 | import { execute } from 'web-threads'
|
59 |
|
60 | function Func(value){
|
61 | this.value = value
|
62 | }
|
63 | Func.prototype.foo = function(otherValue){
|
64 | return this.value * otherValue
|
65 | };
|
66 | var instance = new Func(2)
|
67 | let params = {
|
68 | fn: instance.foo,
|
69 | context: instance,
|
70 | args: [4]
|
71 | }
|
72 | execute(params)
|
73 | .then(console.log)
|
74 | .catch(console.error)
|
75 | ```
|
76 |
|
77 | ### ES6 class call function with arguments
|
78 |
|
79 | ```js
|
80 | import { execute } from 'web-threads'
|
81 |
|
82 | class someClass {
|
83 | constructor(val){
|
84 | this.val = val
|
85 | }
|
86 | foo(some){
|
87 | return this.val * some
|
88 | }
|
89 | }
|
90 |
|
91 | var instance = new someClass(2)
|
92 | let params = {
|
93 | fn: instance.foo,
|
94 | context: instance,
|
95 | args: [4]
|
96 | }
|
97 | execute(params)
|
98 | .then(console.log)
|
99 | .catch(console.error)
|
100 | ```
|
101 | #### Inspired in:
|
102 | - [work done pairing with @kmruiz for ui.wind.js](https://github.com/CodeInBrackets/ui.wind.js)
|
103 | - [scottlogic.com post](https://blog.scottlogic.com/2011/02/24/web-workers-part-3-creating-a-generic-worker.html)
|
104 | - [@vkiryukhin](http://www.eslinstructor.net/vkthread/)
|
105 |
|
106 |
|
107 | ##### Web graphic by <a href="http://www.flaticon.com/authors/picol">picol</a> from <a href="http://www.flaticon.com/">Flaticon</a> is licensed under <a href="http://creativecommons.org/licenses/by/3.0/" title="Creative Commons BY 3.0">CC BY 3.0</a>. Check out the new logo that I created on <a href="http://logomakr.com" title="Logo Makr">LogoMakr.com</a> https://logomakr.com/09u4Zz
|