1 | # Webform Toolkit
|
2 |
|
3 | [](https://badge.fury.io/js/webform-toolkit) [](https://www.npmjs.com/package/webform-toolkit) [](https://app.travis-ci.com/github/nuxy/webform-toolkit) [](https://packagephobia.com/result?p=webform-toolkit) [](https://github.com/nuxy/webform-toolkit/releases)
|
4 |
|
5 | Create a HTML form with field validation and custom errors.
|
6 |
|
7 | 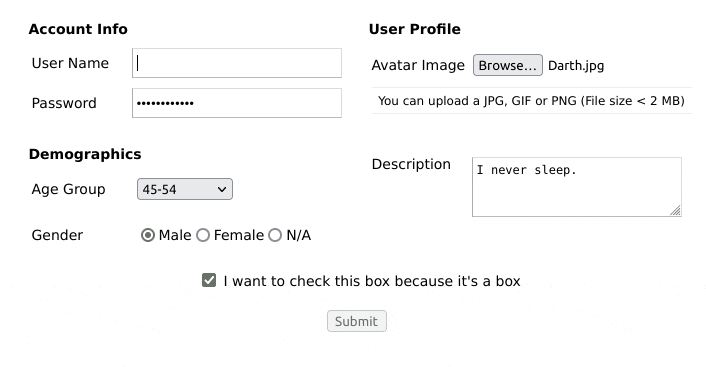
|
8 |
|
9 | ## Features
|
10 |
|
11 | - Extensible HTML/CSS interface.
|
12 | - Compatible with all modern desktop and mobile web browsers.
|
13 | - Easy to set-up and customize.
|
14 | - Provides form input validation using REGEX ([regular expressions](http://www.regular-expressions.info/reference.html))
|
15 | - Supports synchronous form-data POST
|
16 | - Supports FORM submit callback for custom AJAX handling.
|
17 | - Supports dynamic ([on the fly](#adding-fields)) field creation.
|
18 |
|
19 | ## Dependencies
|
20 |
|
21 | - [Node.js](https://nodejs.org)
|
22 |
|
23 | ## Installation
|
24 |
|
25 | Install the package into your project using [NPM](https://npmjs.com), or download the [sources](https://github.com/nuxy/webform-toolkit/archive/master.zip).
|
26 |
|
27 | $ npm install webform-toolkit
|
28 |
|
29 | ## Usage
|
30 |
|
31 | There are two ways you can use this package. One is by including the JavaScript/CSS sources directly. The other is by importing the module into your component.
|
32 |
|
33 | ### Script include
|
34 |
|
35 | After you [build the distribution sources](#cli-options) the set-up is fairly simple..
|
36 |
|
37 | ```html
|
38 | <script type="text/javascript" src="path/to/webform-toolkit.min.js"></script>
|
39 | <link rel="stylesheet" href="path/to/webform-toolkit.min.css" media="all" />
|
40 |
|
41 | <script type="text/javascript">
|
42 | webformToolkit(container, settings, callback);
|
43 | </script>
|
44 | ```
|
45 |
|
46 | ### Module import
|
47 |
|
48 | If your using a modern framework like [Aurelia](https://aurelia.io), [Angular](https://angular.io), [React](https://reactjs.org), or [Vue](https://vuejs.org)
|
49 |
|
50 | ```javascript
|
51 | import WebFormToolkit from 'webform-toolkit';
|
52 | import 'webform-toolkit/dist/webform-toolkit.css';
|
53 |
|
54 | const webformToolkit = new WebformToolkit(container, settings, callback);
|
55 | ```
|
56 |
|
57 | ### HTML markup
|
58 |
|
59 | ```html
|
60 | <div id="webform-toolkit"></div>
|
61 | ```
|
62 |
|
63 | ### Example
|
64 |
|
65 | ```javascript
|
66 | const settings = {
|
67 | action: 'https://www.domain.com/handler',
|
68 | params: 'name1=value1&name2=value2',
|
69 | groups: [
|
70 | {
|
71 | legend: 'Login Form',
|
72 | fields: [
|
73 | {
|
74 | id: 'username',
|
75 | label: 'User Name',
|
76 | type: 'text',
|
77 | name: 'username',
|
78 | value: null,
|
79 | maxlength: 15,
|
80 | filter: '^\\w{0,15}$',
|
81 | description: null,
|
82 | placeholder: null,
|
83 | error: 'Supported characters: A-Z, 0-9 and underscore',
|
84 | required: true
|
85 | },
|
86 | {
|
87 | id: 'password',
|
88 | label: 'Password',
|
89 | type: 'password',
|
90 | name: 'password',
|
91 | value: null,
|
92 | maxlength: 15,
|
93 | filter: '^(?!password)(.{0,15})$',
|
94 | description: null,
|
95 | placeholder: null,
|
96 | error: 'The password entered is not valid',
|
97 | required: true
|
98 | }
|
99 | ]
|
100 | }
|
101 | ]
|
102 | };
|
103 |
|
104 | const container = document.getElementById('webform-toolkit');
|
105 |
|
106 | const webformToolkit = new WebformToolkit(container, settings, callback);
|
107 | ```
|
108 |
|
109 | ## Field definitions
|
110 |
|
111 | | Attribute | Description | Required |
|
112 | |-------------|---------------------------------------------------------------------------------------------------|----------|
|
113 | | id | Field ID value. | true |
|
114 | | label | Field label value. | true |
|
115 | | type | Supported types (`text`, `hidden`, `password`, `checkbox`, `radio`, `file`, `select`, `textarea`) | true |
|
116 | | name | Form element name. | true |
|
117 | | value | Default value. | false |
|
118 | | maxlength | Input type maximum length. | false |
|
119 | | filter | Validate form input using REGEX | false |
|
120 | | description | Custom field description. | false |
|
121 | | placeholder | Input field type placeholder text. | false |
|
122 | | error | Custom error message (Required, if `filter` is defined) | false |
|
123 | | required | Required field. | false |
|
124 |
|
125 | ## Callback processing
|
126 |
|
127 | When a callback function is defined a form object is returned. This allows you to define a custom AJAX handler based on the requirements of your application. The following function corresponds to the [example](#usage) provided above.
|
128 |
|
129 | ```javasctipt
|
130 | function callback(form) {
|
131 | const xhr = new XMLHttpRequest();
|
132 |
|
133 | xhr.addEventListener('load', function() {
|
134 | if (this.status == 200) {
|
135 | alert(response);
|
136 | }
|
137 | });
|
138 |
|
139 | xhr.open('POST', form.getAttribute('action'));
|
140 | xhr.send(new FormData(form));
|
141 | }
|
142 | ```
|
143 |
|
144 | ## Adding fields
|
145 |
|
146 | I have added a method to dynamically create form fields that can be added to an existing webform. An optional callback has also been provided to for post-processing FORM and field elements. This makes it easy to show/hide fields using conditions and expressions.
|
147 |
|
148 | ```javascript
|
149 | webformToolkit.create({
|
150 | id: 'new_field_id',
|
151 | label: 'New Field',
|
152 | type: 'text',
|
153 | name: 'new_field',
|
154 | value: null,
|
155 | maxlength: null,
|
156 | filter: '^[a-zA-Z0-9_]{0,255}$',
|
157 | description: 'This is my new field',
|
158 | placeholder: null,
|
159 | error: 'Supported characters: A-Z, 0-9 and underscore',
|
160 | required: true
|
161 | },
|
162 | function(form, elm) {
|
163 | form.appendChild(elm); // default: last in fieldset
|
164 | });
|
165 | ```
|
166 |
|
167 | ## Best practices
|
168 |
|
169 | Just because you are filtering form input on the client-side is NO EXCUSE to not do the same on the server-side. Security is a two-way street, and BOTH ends should be protected.
|
170 |
|
171 | ## Developers
|
172 |
|
173 | ### CLI options
|
174 |
|
175 | Run [ESLint](https://eslint.org) on project sources:
|
176 |
|
177 | $ npm run lint
|
178 |
|
179 | Transpile ES6 sources (using [Babel](https://babeljs.io)) and minify to a distribution:
|
180 |
|
181 | $ npm run build
|
182 |
|
183 | Run [WebdriverIO](https://webdriver.io) E2E tests:
|
184 |
|
185 | $ npm run test
|
186 |
|
187 | ## Unsupported releases
|
188 |
|
189 | To install deprecated versions use [Bower](http://bower.io) or download the package [by tag](https://github.com/nuxy/webform-toolkit/tags).
|
190 |
|
191 | ### v3 (no dependencies)
|
192 |
|
193 | $ bower install webform-toolkit#2
|
194 |
|
195 | ### v2 (requires [jQuery 1.8.3](http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js))
|
196 |
|
197 | Compatible with Firefox 3.6, Chrome, Safari 5, Opera, and Internet Explorer 7+ web browsers.
|
198 |
|
199 | $ bower install webform-toolkit#1
|
200 |
|
201 | ## License and Warranty
|
202 |
|
203 | This package is distributed in the hope that it will be useful, but without any warranty; without even the implied warranty of merchantability or fitness for a particular purpose.
|
204 |
|
205 | _webform-toolkit_ is provided under the terms of the [MIT license](http://www.opensource.org/licenses/mit-license.php)
|
206 |
|
207 | ## Author
|
208 |
|
209 | [Marc S. Brooks](https://github.com/nuxy)
|